はじめに
今回は、↓のシートのようにMenuを追加する方法をお伝えします。

コード例 メニューを追加する
まず、メニューを追加します。
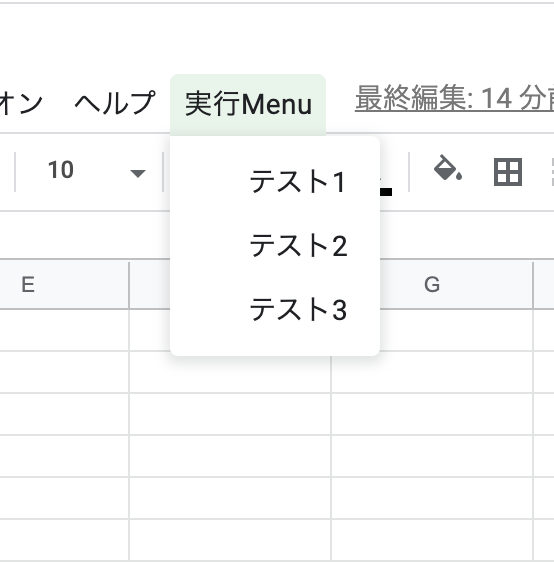
/** * 起動時に実行する */ function onOpen() { let ui = SpreadsheetApp.getUi(); ui.createMenu("実行Menu") // 1 実行Menuをクリックする。 .addItem("テスト1", "test1") // テスト1 をクリックすると test1 関数を実行する。 .addItem("テスト2", "test2") // テスト2 をクリックすると test2 関数を実行する。 .addItem("テスト3", "test3") // テスト3 をクリックすると test3 関数を実行する。 .addToUi() }
コード例2 メニュー+サブメニューを追加する
次は、↓の動画のようなMenuとサブメニュー形式でメニューを作成します。
/** * 起動時に実行する */ function onOpen() { let ui = SpreadsheetApp.getUi(); ui.createMenu("実行Menu") // 1 実行Menuをクリックする。 .addSubMenu(ui.createMenu("サブメニュー1") // 2 サブメニュー1 をクリックすると、 下層のメニューを表示する。 .addItem("テスト1-1", "test1-1") //テスト1-1 をクリックすると、test1-1 関数を実行する。 .addItem("テスト1-2", "test1-2") //テスト1-2 をクリックすると、test1-2 関数を実行する。 .addItem("テスト1-3", "test1-3") //テスト1-3 をクリックすると、test1-3 関数を実行する。 ) .addSubMenu(ui.createMenu("サブメニュー2") // 2 サブメニュー2 をクリックすると、 下層のメニューを表示する。 .addItem("テスト2-1", "test2-1") //テスト2-1 をクリックすると、test2-1 関数を実行する。 .addItem("テスト2-2", "test2-2") //テスト2-2 をクリックすると、test2-2 関数を実行する。 .addItem("テスト2-3", "test2-3") //テスト2-3 をクリックすると、test2-3 関数を実行する。 ) .addSubMenu(ui.createMenu("サブメニュー3") // 2 サブメニュー3 をクリックすると、 下層のメニューを表示する。 .addItem("テスト3-1", "test3-1") //テスト3-1 をクリックすると、test3-1 関数を実行する。 .addItem("テスト3-2", "test3-2") //テスト3-2 をクリックすると、test3-2 関数を実行する。 .addItem("テスト3-3", "test3-3") //テスト3-3 をクリックすると、test3-3 関数を実行する。 ) .addToUi() //メニューをセットする。 }
Custom Menuについて
今回使用したClassとMethodは、Custom Menuといいます。スプレッドシートの画面上に自由にボタンを作成することができます。
Scripts can extend certain Google products by adding user-interface elements that, when clicked, execute an Apps Script function. The most common example is running a script from a custom menu item in Google Docs, Sheets, Slides, or Forms, but script functions can also be triggered by clicking on images and drawings in Google Sheets.
https://developers.google.com/apps-script/guides/menus
スクリプトは、クリックするとApps Script関数を実行するユーザーインターフェース要素を追加して、特定のGoogleサービスを拡張できます。最も一般的な例は、Googleドキュメント、スプレッドシート、スライド、フォームのカスタムメニューアイテムからスクリプトを実行することですが、スクリプト機能は、Googleスプレッドシートの画像や図形をクリックしてトリガーすることもできます。
今回使用したClassとMethodのリファレンス情報
Ui Class
An instance of the user-interface environment for a Google App that allows the script to add features like menus, dialogs, and sidebars. A script can only interact with the UI for the current instance of an open editor, and only if the script is container-bound to the editor.
スクリプトがメニュー、ダイアログ、サイドバーなどの機能を追加できるようにするGoogleアプリのユーザーインターフェース環境のインスタンス。スクリプトは、開いているエディターの現在のインスタンスのUIとのみ対話でき、スクリプトがエディターにコンテナーバインドされている場合のみです。
https://developers.google.com/apps-script/reference/base/ui?hl=ja
createMenu(caption)
Creates a builder that can be used to add a menu to the editor’s user interface. The menu isn’t actually be added until Menu.addToUi() is called. For more information, see the guide to menus. The label for a top-level menu should be in headline case (all major words capitalized), although the label for a sub-menu should be in sentence case (only the first word capitalized). If the script is published as an add-on, the caption parameter is ignored and the menu is added as a sub-menu of the Add-ons menu, equivalent to createAddonMenu().
https://developers.google.com/apps-script/reference/base/ui?hl=ja#createmenucaption
エディターのユーザーインターフェイスにメニューを追加するために使用できるビルダーを作成します。 Menu.addToUi()が呼び出されるまで、メニューは実際には追加されません。詳細については、メニューのガイドを参照してください。トップメニューのラベルは見出しの大文字(すべての主要な単語は大文字)にする必要がありますが、サブメニューのラベルは文の大文字(最初の単語のみ大文字)にする必要があります。スクリプトがアドオンとして公開されている場合、captionパラメーターは無視され、メニューはcreateAddonMenu()と同等のアドオンメニューのサブメニューとして追加されます。
参考:onOpen()関数とは
onOpen()関数は、シンプルトリガー の一つで「起動」を発動条件としたトリガーです。ユーザーがスプレッドシートを開いたタイミングで実行するため、メニューに追加に適しています。
The
onOpen(e)
trigger runs automatically when a user opens a spreadsheet, document, presentation, or form that they have permission to edit. (The trigger does not run when responding to a form, only when opening the form to edit it.)onOpen(e)
is most commonly used to add custom menu items to Google Sheets, Slides, Docs, or Forms.onOpen(e)トリガーは、ユーザーが編集権限を持つスプレッドシート、ドキュメント、プレゼンテーション、またはフォームを開くと自動的に実行されます。 (トリガーは、フォームに応答するときは実行されません。フォームを開いて編集するときのみ実行されます。)onOpen(e)は、Googleスプレッドシート、スライド、ドキュメント、またはフォームにカスタムメニュー項目を追加するために最もよく使用されます。
https://developers.google.com/apps-script/guides/triggers
*なお、他の関数名で起動時にメニューを設置する場合は、インストールトリガーにより設定する必要があります。
コメント